Components of Px Framework:
Validation, checking of the entered values by means
of the PxWebQuery component and other visual components (PxEdit, PxComboBox, etc.)
Through the PxEdit component (as well as PxComboBox, PxFlyComboBox, PxJSDatePicker, etc.)
the PxWebQuery component contains and automatic validation. If the table column
linked to the PxEdit component is of the
string type, validation of the string
length is automatically carried out, if it is of the
int type, validation
operation to check whether the integer has been entered is carried out,
in case of the
date type, validation operation to check whether the valid
date has been entered is carried out, etc.
The picture below shows an example where we have entered a string which length
exceeded the length of string defined in the database. Then we have
entered an invalid date.
Linking the PxWebQuery and the PxEdit component causes the automatic validation.
If the value specified in the PxEdit component does not correspond to the given type,
it shall not pass the automatic validation, data storing shall be automatically
stopped, and the PxEdit component shall report an error message stating
the reason for which the data has not been stored.
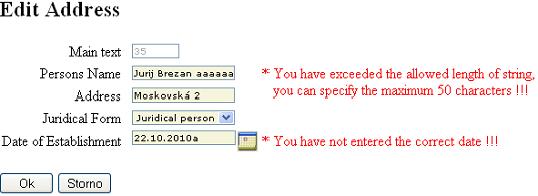
In addition to the automatic validation, there are additional validations,
defined in the PxWebQuery component via the AddParamValidation parameter.
wquAdresar.AddParamValidation("name", "vtIsNotNull");
In this case, data storing shall not be carried out if the "Name" column
is not filled out, and after pressing the "Ok" button the original form shall
remain and the "Persons Name" component shall report the error message.
Please refer to the picture below:
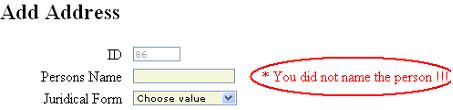
Similarly, if the "vtIsNotNull" validation parameter is applied to the "juridical form" column,
the original form shall remain after pressing the "Ok" button until any value
from the "juridical form" list is selected. The command specifying the juridical form
validation parameter shall be as follows:
wquAdresar.AddParamValidation("idpravnaforma", "vtIsNotNull");
If no Juridical Form from the combobox is selected, the original form shall remain
after the "Ok" button is pressed, and the reason why the form has not been saved
shall be stated in red letters. Please refer to the picture below.
Definition of the AddParamValidation command:
public void AddParamValidation(string sFieldName, string sEnumValidationTest,
string sRegularExpression, Int32 iMinMaxValue, string sErrorMsg)
public void AddParamValidation(string sFieldName, string sEnumValidationTest,
Int32 iMinMaxValue)
public void AddParamValidation(string sFieldName, string sEnumValidationTest)
Description of the parameters of the AddParamValidation command:
sFieldName - field name , column name, to which this validation type shall be applied
sEnumValidationTest - validation type, control type that shall be carried out, description of individual fields shall be given below
sRegularExpression - expression for setting the validation via regular expressions
iMinMaxValue - minimal, maximal expression value at the specified integer field type
sErrorMsg - this expression serves for re-writing or entering of a new error message at this validation type
Description of individual validation types:
Type validation(sEnumValidationTest) | Description of the validation type
|
vtIsNotNull | This type checks whether any value is specified, if none value is specified, the error message "No value is specified !!!" shall be sent
|
vtValidToEmail | This type checks whether valid e-mail address is specified
|
vtValidToMaxNumber | This type checks whether the smaller number than in the iMinMaxValue variable is specified, if not, the error message is sent
|
vtValidToMinNumber | This type checks whether the bigger number than in the iMinMaxValue variable is specified, if not, the error message is sent
|
vtValidToPSC | This type checks whether the valid zip code is specified, if not, the error message is sent
|
vtValidToRegularExpression | This type checks whether the specified value correspond to the set regular expression , if not, the error message is sent
|
vtValidToString | This type checks whether the specified string contains only letters, if not, the error message is sent
|
vtValidToTelefon | This type checks whether the valid phone number is specified, if not, the error message is sent
|
vtValidToURL | This type checks whether the valid URL value is specified, if not, the error message is sent
|
vtValidToCurrency | This type checks whether the, the valid currency type number is specified, if not, the error message is sent
|
vtValidToNumber | This type checks whether the, the whole specified number is valid, if not, the error message is sent
|
vtValidToFloat | This type checks whether the, valid real number is specified, if not, the error message is sent
|
vtValidToDateTime | This type checks whether the, specified expression stands for the valid date
|
vtValidToLengthString | This type checks is the length of the string value of the variable iMinMaxValue, if string length is greater than the length of the variable iMinMaxValue return an error message
|
vtIsUnique | This type checks whether the value is unique, unique for the particular column
|
Each type of validation has the fixed error message assigned to it.
If you want to change this error message in case its text is not satisfactory,
you can do it in the following way:
wquAdresar.AddParamValidation("name", "vtIsNotNull", "", 0, "You did not name the person!!!");
Further, there is an example of validation using regular expressions.
This book is not intended to describe how to operate with regular expressions and how to compile them, for further information please refer to various web resources.
We have added another column to the "adresar" table. This column serves for entering the zip code.
Define validation via the regular expression to the Adresar.aspx.cs file as shown below:
string sRegVyrazToPSC = @"^\d{5}$|^\d{3}\s\d{2}$";
wquAdresar.AddParamValidation("psc", "vtValidToRegularExpression", sRegVyrazToPSC, 0, "You have not entered a valid zipcode !!!");
The defined regular expression allows to enter a 5 digit number, either
with a space after the third digit, with or without a gap. Whenever you want
to carry out validation via the regular expression, you have to specify
the "vtValidToRegularExpresion" validation type. If validation for the zip code
item is defined in such a way, entering of an incorrect zip code shall stop saving of a form.
The error message defined in the "ERRORMSG" expression shall appear after
the zip code component, please refer to the picture below.
During the automatic validation and control of the length string, validation may not work quite right.
It depends on the code page that is set in the database. If you use the database
code page with UTF8 the characters with diacritics in the Oracle database occupy
the 2 characters and some special characters to 3 characters in the database.
Therefore, you must set the property of the
CharacterCode component in PxWebQuery to "UTF8"
so that the validation of the string length work properly. In the following we list costants
of the
CharacterCode property for the proper functionality of validation of the string length.
The
CharacterCode property is default set to ASCII.
N. | Constant CharacterCode
|
1 | ASCII
|
2 | UTF8
|
3 | UTF16
|
4 | UTF32
|
5 | UTF7
|
If we set the code page to UTF8 in the database we should set it to the CharacterCode
property before opening the PxWebQuery component. See examples below:
wquAdresar.CharacterCode = "UTF8";
wquAdresar.Open();
...
Here is a functional example of the PxWebQuery component, even with source code.
This functional example on this site runs on MySQL 5.0 database.
Others articles of Px Framework:
- PxWebQuery - component for working with databases
- PxSuperGrid - component for the direct display of data in the table
- PxEdit - component for data editing, similar to the TextBox component
- PxComboBox - component for selecting data from a list, similar to the DropDownList component
- PxCheckBox - component for checking the value (Check / UnCheck value)
- PxFlyComboBox - set of the consecutively linked comboboxes, suitable for the work with structured data (for example: selection of category and subcategory)
- PxGreatRepeater - component for entering data with repeating structure, maximum number of values is limited
- PxJSDatePicker - component for the date entry, based on the JavaScript
- PxDbNavigator - the component for the work with the PxWebQuery components, row cursor movement, etc.
- PxLabel - component for data display
- PxCheckBoxList - component to view and select values from the list
- PxRadioButtonList - component for view and selection of a value from the list
- PxChart - the component for displaying and working with charts
- PxFilterView - visual component for filtering the table data contents in the PxWebQuery component
- PxUploader - component for uploading binary and text files to the server
- PxLogin - component for authorization and logging into the application
- Data loading from the Oracle, MSSQL, MySQL, FireBird, Interbase database by means of the PxWebQuery components
- Program inserting, editing or deleting of row into the database by means of the PxWebQuery component
- Loading values from the PxWebQuery component via the while cycle
- Row search in the PxWebQuery component according to the value entered and the name of the column where the search shall be carried out
- The "ReOpen" procedure of the PxWebQuery component and data re-load into the PxWebQuery component
- Validation, checking of the entered values by means of the PxWebQuery component and other visual components (PxEdit, PxComboBox, etc.)
- Events of the PxWebQuery component
- A special function GetValueFromStructKey of the PxWebQuery component
- Setting the language mutation of the Px Framework
- Finding the current version of the Px Framework
It doesn't the intention of this part website, describe in detail the work with PxFramework components, a detailed description of the component
available in manual, which can be downloaded here:
Download manual of Px Framework